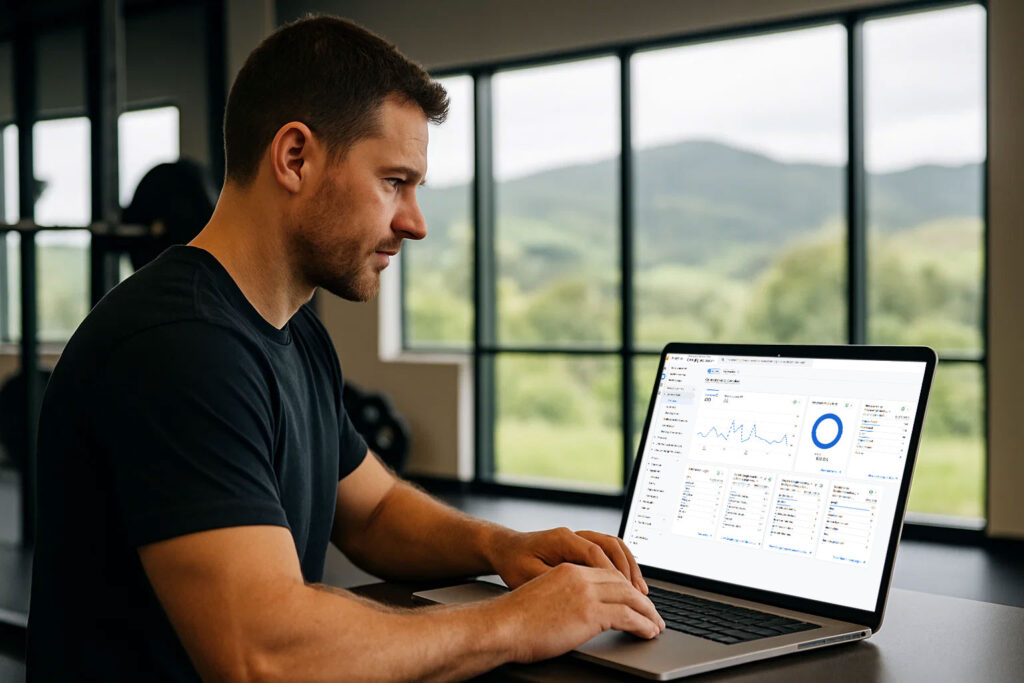
Skyrocketing Google Ads Costs: What You Need to Know in 2025
If you’re a business owner investing money into a Google Ads campaign, you’ve likely noticed your ad spend creeping up without always seeing the results
If you’re a business owner investing money into a Google Ads campaign, you’ve likely noticed your ad spend creeping up without always seeing the results
Website design, Elementor build, and now ongoing hosting and maintenance. We’re pleased to welcome AG Compliance Solutions to the Creative Digital family—this time as a
Tracking external link clicks is crucial for understanding how visitors interact with your website. Whether users are clicking on outbound links to partner sites, affiliate
Having a professional website is essential for any business. However, many small businesses, startups, and entrepreneurs assume that web design comes with a hefty price
We’re very excited to welcome Balex Marine to our premium website hosting and maintenance services at Creative Digital. Balex Marine, renowned for their innovative automatic
Starting a new website project can be an exciting but overwhelming process. Before contacting a web designer for a quotation, it’s helpful to explore what